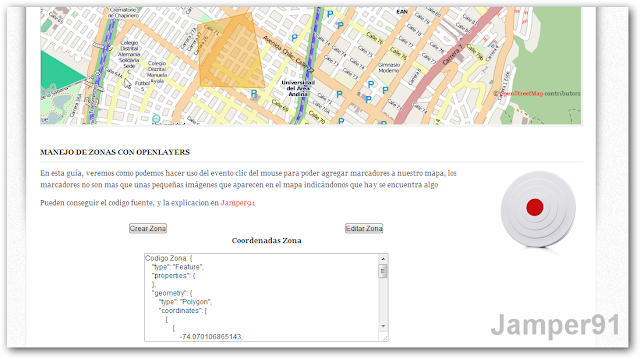
En esta guía, veremos como podemos crear sobre el mapa de forma dinamia "zonas". Estas zonas son áreas que se muestran con otro color sobre el mapa, que delimitan lo que a nosotros nos apetece, pueden ser "zonas de cuidado", "areas de cobertura", etc.
Para poder crear estas zonas, debemos añadir una capa nueva al mapa, esta capa sera del tipo Vector
vectors = new OpenLayers.Layer.Vector("Capa Vectorial"); mapa.addLayer(vectors);
También se usaran 2 variables para determinar que acción se va a realizar:
- drawP: si dibujar una nueva área
- editP: editar una ya existente
drawP=new OpenLayers.Control.DrawFeature(vectors,OpenLayers.Handler.Polygon) mapa.addControl(drawP); editP= new OpenLayers.Control.ModifyFeature(vectors); mapa.addControl(editP);
En este caso, se instancia la variable drawP, para que pintara un polígono, pero también se puede escoger Point o RegularPolygon (para ver mas información sobre la clase OpenLayers.Handler, visitar la documentación oficial ).
var options = { hover: true, onSelect: serialize }; select = new OpenLayers.Control.SelectFeature(vectors, options); mapa.addControl(select);
En esta sección, lo que se hace es agregar un control al evento "SelectFeature", esto con el fin de que cuando se coloque el mouse sobre un área (hover), se pinte su código en el cuadro de dialogo. Para realizar estas acciones se llama a la función "serialize", tal como se describe en la variable options, que se pasa al constructor de la variable select.
La funcion updateFormats es usada para determinar en que formato se mostrara la salida, con formato me refieron a las "medidas " de coordenadas.
Las funciones activarDrawFeacture y activarModifyFeacture son usadas para determina que accion a realizar, si pintar una zona (feature) o modificar una ya existente, para esto, solo basta con llamar dichas funciones desde los botones respectivos.
Para poder realizar todas las acciones anteriomente mencionadas, primero se debe llamar a la funcion iniciarDrawFeacture, que se encarga de iniciar todo lo necesario (capas, variables,controles,etc). Para hacer esto, lo realizamos desde la funcion onLoad del body
<body onload="iniciar(); iniciarDrawFeacture();">
Aqui esta el codigo de la pagina zonas.html
<html xmlns="http://www.w3.org/1999/xhtml"> <head> <script type="text/javascript" src="http://code.jquery.com/jquery-2.0.3.min.js"></script> <script type="text/javascript" src="http://www.openlayers.org/api/OpenLayers.js"></script> <script type="text/javascript" src="js/OpenLayers_base.js"></script> <script type="text/javascript" src="js/OpenLayers_features.js"></script> <style type="text/css"> .smallmap { width: 929px; height: 300px; } </style> </head> <body <body onLoad="iniciar();iniciarDrawFeacture();"> <h3>Nuestro primer mapa con OpenLayers</h3> <div id="mapa" class="smallmap"> </div> <table width="80%"> <tr> <td align="center"> <input type="submit" name="crearFeature" id="crearFeature" value="Crear Zona" onclick="activarDrawFeacture()" /> </td> <td align="center"> <input type="submit" name="editarFeature" id="editarFeature" value="Editar Zona" onclick="activarModifyFeacture()" /> </td> </tr> </table> <p align="center"><strong>Coordenadas Zona</strong></p> <div align="center"> <textarea name="coordenadasZona" cols="80" rows="10" id="coordenadasZona"></textarea> </input> </body> </html>
Aqui esta el archivo OpenLayers_features.js
var drawP,editP,vectors,select,formats; //Funcion se encarga de agregar los controles para poder dibujar y editar polygonos function iniciarDrawFeacture() { vectors = new OpenLayers.Layer.Vector("Capa Vectorial"); mapa.addLayer(vectors); drawP=new OpenLayers.Control.DrawFeature(vectors,OpenLayers.Handler.Polygon) mapa.addControl(drawP); editP= new OpenLayers.Control.ModifyFeature(vectors); mapa.addControl(editP); updateFormats(); var options = { hover: true, onSelect: serialize }; select = new OpenLayers.Control.SelectFeature(vectors, options); mapa.addControl(select); } //Esta funcion permite dibujar un feature() function activarDrawFeacture() { editP.deactivate(); drawP.activate(); select.activate(); } //Esta funcion permite obtener el codigo de un poligono y mostrarlo en un string function serialize(feature) { var type = "geojson"; // second argument for pretty printing (geojson only) var pretty = 1; var str = formats['out'][type].write(feature, pretty); // not a good idea in general, just for this demo str = str.replace(/,/g, ', '); $("#coordenadasZona").attr('value','Codigo Zona: '+str); return str; } //Esta funcion es para tener unos formatos estandar de codificacion function updateFormats() { var in_options = { 'internalProjection': mapa.baseLayer.projection, 'externalProjection': new OpenLayers.Projection("EPSG:4326") }; var out_options = { 'internalProjection': mapa.baseLayer.projection, 'externalProjection': new OpenLayers.Projection("EPSG:4326") }; var gmlOptions = { featureType: "feature", featureNS: "http://example.com/feature" }; var gmlOptionsIn = OpenLayers.Util.extend( OpenLayers.Util.extend({}, gmlOptions), in_options ); var gmlOptionsOut = OpenLayers.Util.extend( OpenLayers.Util.extend({}, gmlOptions), out_options ); var kmlOptionsIn = OpenLayers.Util.extend( {extractStyles: true}, in_options); formats = { 'in': { wkt: new OpenLayers.Format.WKT(in_options), geojson: new OpenLayers.Format.GeoJSON(in_options), georss: new OpenLayers.Format.GeoRSS(in_options), gml2: new OpenLayers.Format.GML.v2(gmlOptionsIn), gml3: new OpenLayers.Format.GML.v3(gmlOptionsIn), kml: new OpenLayers.Format.KML(kmlOptionsIn), atom: new OpenLayers.Format.Atom(in_options), gpx: new OpenLayers.Format.GPX(in_options), encoded_polyline: new OpenLayers.Format.EncodedPolyline(in_options) }, 'out': { wkt: new OpenLayers.Format.WKT(out_options), geojson: new OpenLayers.Format.GeoJSON(out_options), georss: new OpenLayers.Format.GeoRSS(out_options), gml2: new OpenLayers.Format.GML.v2(gmlOptionsOut), gml3: new OpenLayers.Format.GML.v3(gmlOptionsOut), kml: new OpenLayers.Format.KML(out_options), atom: new OpenLayers.Format.Atom(out_options), gpx: new OpenLayers.Format.GPX(out_options), encoded_polyline: new OpenLayers.Format.EncodedPolyline(out_options) } }; } function activarModifyFeacture() { editP.activate(); drawP.deactivate(); select.deactivate(); editP.mode = OpenLayers.Control.ModifyFeature.RESHAPE; editP.createVertices = true; }
Si desean el código
fuente completo, con un buen diseño, pueden visitar el siguiente link
0 comentarios :
Publicar un comentario